Scaling Web Infrastructure with CDNs
Optimize website performance and reliability by utilizing Content Delivery Networks (CDNs) for global content distribution, ensuring fast and accessible web experiences.
In today’s digital landscape, delivering content quickly and efficiently to users across the globe is crucial for any successful web application. Content Delivery Networks (CDNs) have emerged as a powerful solution to this challenge, offering improved performance, reduced latency, and enhanced scalability. In this blog, we’ll explore how CDNs can help scale your web infrastructure, discuss their benefits, and provide practical examples to get you started.
What is a CDN?
A Content Delivery Network (CDN) is a geographically distributed network of servers that work together to provide fast delivery of Internet content. CDNs store cached versions of your website content in multiple locations around the world, allowing users to access the content from the server nearest to them.
How CDNs Work
Content Caching: The CDN caches static content from your origin server.
User Request: When a user requests content, their request is routed to the nearest CDN server.
Content Delivery: The CDN server delivers the cached content to the user.
Cache Update: If the content isn’t in the cache or has expired, the CDN fetches it from the origin server and updates its cache.
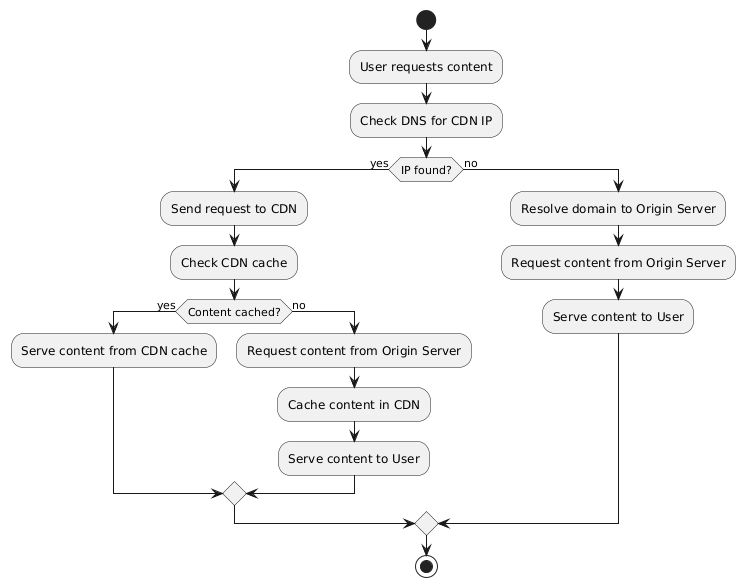
Why Use a CDN?
Improved Performance
- Faster Load Times: By serving content from geographically closer servers, CDNs reduce latency and improve page load times.
Reduced Server Load
- Offload Traffic: CDNs handle a significant portion of your traffic, reducing the load on your origin servers.
High Availability and Redundancy
- Distributed Network: CDNs provide built-in redundancy, ensuring your content remains available even if one server goes down.
Enhanced Security
- DDoS Protection: Many CDNs offer built-in protection against Distributed Denial of Service (DDoS) attacks.
Global Reach
- Expand Your Audience: CDNs make it easier to deliver content to a global audience with consistent performance.
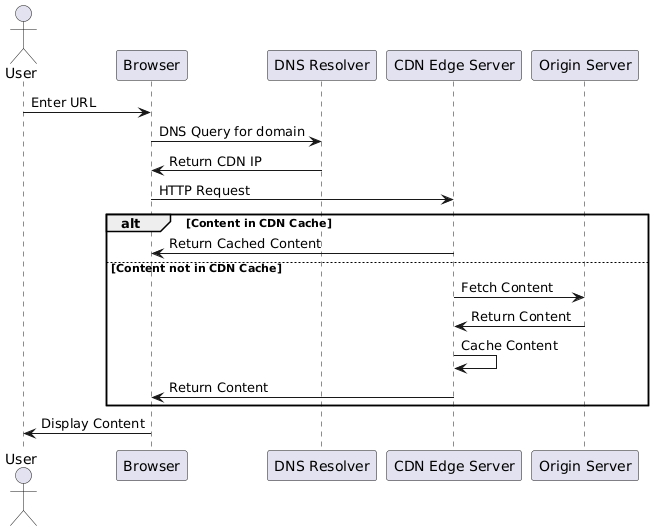
Implementing a CDN with Cloudflare
Let’s walk through a practical example of implementing a CDN using Cloudflare, one of the popular CDN providers.
Step 1: Sign Up for Cloudflare
First, create an account on Cloudflare and add your website.
Step 2: Update DNS Settings
Cloudflare will provide you with new nameservers. Update your domain’s nameservers at your domain registrar to point to Cloudflare’s nameservers.
Step 3: Configure Cloudflare Settings
In your Cloudflare dashboard, you can configure various settings. Here’s an example of how to enable caching for static content:
- Go to the “Caching” tab in your Cloudflare dashboard.
- Enable “Caching Level” and set it to “Standard”.
- Set “Browser Cache TTL” to a desired value (e.g., 4 hours).
Step 4: Implement Cloudflare in Your Code
You can optimize your website to work better with Cloudflare by adding some HTTP headers. Here’s an example using Node.js and Express:
const express = require('express');
const app = express();
app.use((req, res, next) => {
// Enable caching for static assets
if (req.url.match(/^\/static/)) {
res.setHeader('Cache-Control', 'public, max-age=3600'); // Cache for 1 hour
}
// Add Cloudflare-specific headers
res.setHeader('CF-Cache-Status', 'DYNAMIC');
next();
});
app.get('/', (req, res) => {
res.send('Hello, CDN-powered World!');
});
app.listen(3000, () => console.log('Server running on port 3000'));
This code adds caching headers for static assets and Cloudflare-specific headers to help with caching and performance optimization.
Here’s a diagram illustrating the flow of requests with Cloudflare CDN:
Advanced CDN Techniques
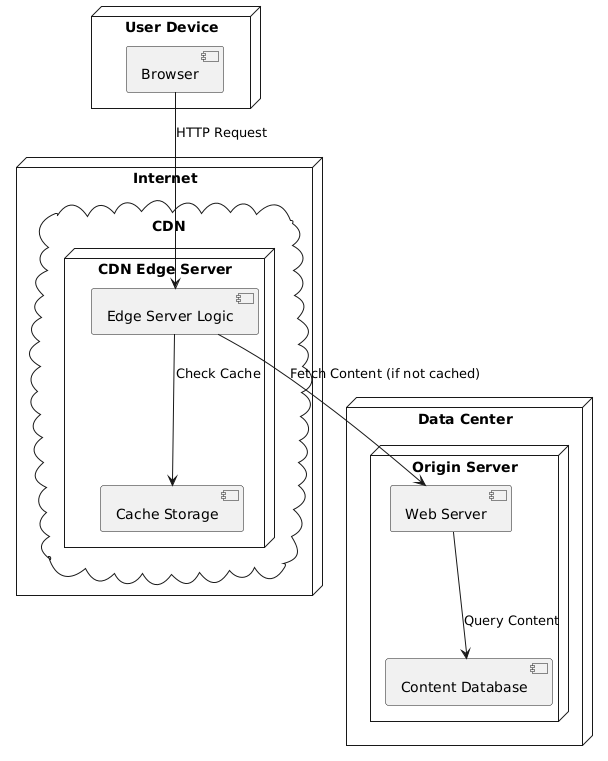
1. Dynamic Content Caching
While CDNs are great for static content, you can also cache dynamic content using techniques like Edge Side Includes ( ESI) or by implementing API caching.
Here’s an example of how to implement API caching with Cloudflare Workers:
addEventListener('fetch', event => {
event.respondWith(handleRequest(event.request))
})
async function handleRequest(request) {
const cacheUrl = new URL(request.url)
const cacheKey = new Request(cacheUrl.toString(), request)
const cache = caches.default
let response = await cache.match(cacheKey)
if (!response) {
response = await fetch(request)
response = new Response(response.body, response)
response.headers.append('Cache-Control', 's-maxage=3600')
event.waitUntil(cache.put(cacheKey, response.clone()))
}
return response
}
This Cloudflare Worker caches API responses for 1 hour, reducing load on your origin server for frequently requested data.
2. Image Optimization
Many CDNs offer on-the-fly image optimization. Here’s an example of how you might use this feature with Cloudflare:
<img src="https://your-website.com/image.jpg?width=800&quality=80" alt="Optimized Image">
By adding query parameters to the image URL, Cloudflare can resize and compress the image on-the-fly, delivering an optimized version to the user.
3. Geolocation Routing
CDNs can also help with serving location-specific content. Here’s an example using Cloudflare Workers:
addEventListener('fetch', event => {
event.respondWith(handleRequest(event.request))
})
async function handleRequest(request) {
const country = request.headers.get('CF-IPCountry')
switch (country) {
case 'US':
return new Response('Hello, America!')
case 'GB':
return new Response('Hello, United Kingdom!')
default:
return new Response('Hello, World!')
}
}
This script serves different content based on the user’s geographic location.
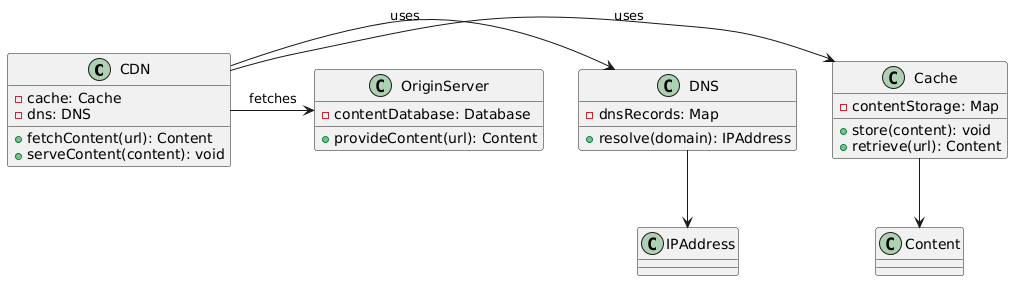
Best Practices for Using CDNs
Choose the Right Content to Cache: Not all content should be cached. Identify static assets that can be safely cached for extended periods.
Set Appropriate TTLs: Time-to-Live (TTL) determines how long content stays in the cache. Set appropriate TTLs based on how frequently your content changes.
Use Cache-Control Headers: Implement proper cache-control headers to give the CDN clear instructions on how to cache your content.
Implement HTTPS: Ensure your CDN supports HTTPS to maintain security for your users.
Monitor Performance: Regularly monitor your CDN’s performance and adjust your configuration as needed.
Wrapping Up
Content Delivery Networks are a powerful tool for scaling web infrastructure, offering improved performance, reduced server load, and enhanced global reach. By implementing a CDN and following best practices, you can significantly improve your website’s performance and user experience.
Whether you’re running a small blog or a large-scale web application, CDNs provide a flexible and efficient solution for delivering content to your users, wherever they may be. As you continue to grow and scale your web infrastructure, remember that CDNs are not just a performance enhancement, but a crucial component of a robust, global web presence.